The Microsoft Dynamics NAV Windows client pages include an all-purpose OnControlAddin Trigger on field controls that control add-ins can invoke to run C/AL code. No code is needed to introduce this event into C/AL. To invoke the OnControlAddIn trigger, a control add-in sends an event to Microsoft Dynamics NAV Server, as shown in the following illustration.
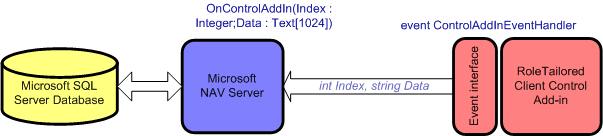
Events allow the control add-in to react to user interaction in the Microsoft Dynamics NAV Windows client. An example of an event is when a user clicks a button in the control add-in display on the page. To program events in a control add-in, you implement the Microsoft.Dynamics.Framework.UI.Extensibility.IEventControlAddInDefinition interface, as shown in the following example.
C# | ![]() |
---|---|
[ControlAddInExport("MyControlAddIn")] public class MyControlAddIn : IEventControlAddInDefinition { ... } |
By firing the ControlAddIn event declared in the base interface, the add-in sends data to Microsoft Dynamics NAV Server in two attributes: Index and Data. The Index attribute value is an integer data type. The Data attribute value is a text string data type. You add C/AL code to the OnControlAddIn trigger to process the data.
![]() | |
---|---|
if (this.ControlAddIn != null) { this.ControlAddIn(100, "Test data"); } |
![]() |
---|
The Data attribute on the OnControlAddIn trigger supports both Text and BigText data types from Microsoft Dynamics NAV Server. The data type is determined by the SourceExpr property of the field control that is applied with the control add-in. If the SourceExpr property value is BigText, then the Data attribute is BigText. Otherwise, the Data attribute is Text[1024]. For more information about data types, see C/AL Functions. |
For more information about how to create events in a control add-in, see How to: Create a Windows Client Control Add-in.
For more information about how to set up the OnControlAddin trigger on a page, see How to: Set Up a Windows Client Control Add-in on a Page.
Exposing Custom Events
To expose a public event in a control add-in, you add the event code to the control add-in class and mark it by using the managed attribute ApplicationVisibleAttribute in the control add-in class.
The following code example is from a control add-in class that exposes a simple method and property.
![]() | |
---|---|
public delegate void TransactionEventHandler(string refNo); [ControlAddInExport("MyControlAddIn")] public class MyControlAddIn : WinFormsControlAddInBase, ... { ... //Exposes an event [ApplicationVisible] public TransactionEventHandler TransactionChanged; ... |
In the example a trigger called OnTransationChanged will be created in the page for the respective field as soon as the add-in is assigned to the field. You can write C/AL code in the trigger, which will be run on the server when the add-in on the client fires the event.